Data science has become an essential part of the business world, with companies relying on data to make informed decisions and gain a competitive edge. As the demand for data professionals continues to rise, learning Python for data analysis has become a valuable skill in the job market. Python is a versatile programming language that offers a wide range of tools and libraries specifically designed for data science. In this blog post, we will explore the basics of Python programming and its importance in data analysis.
Introduction to Data Science
Data science is an interdisciplinary field that combines various techniques and tools from mathematics, statistics, and computer science to extract insights from data. It involves collecting, cleaning, organizing, analyzing, and visualizing data to uncover patterns and trends that can help businesses make data-driven decisions. With the increasing volume of data being generated every day, data science has become crucial for organizations to stay ahead of their competitors.
Python is one of the most popular programming languages used by data scientists due to its simplicity, versatility, and powerful libraries. It provides an easy-to-learn syntax, making it accessible even for beginners. Moreover, it has a vast community of developers continuously working on improving existing libraries and creating new ones, making it a go-to language for data analysis.
Importance of Python in Data Analysis
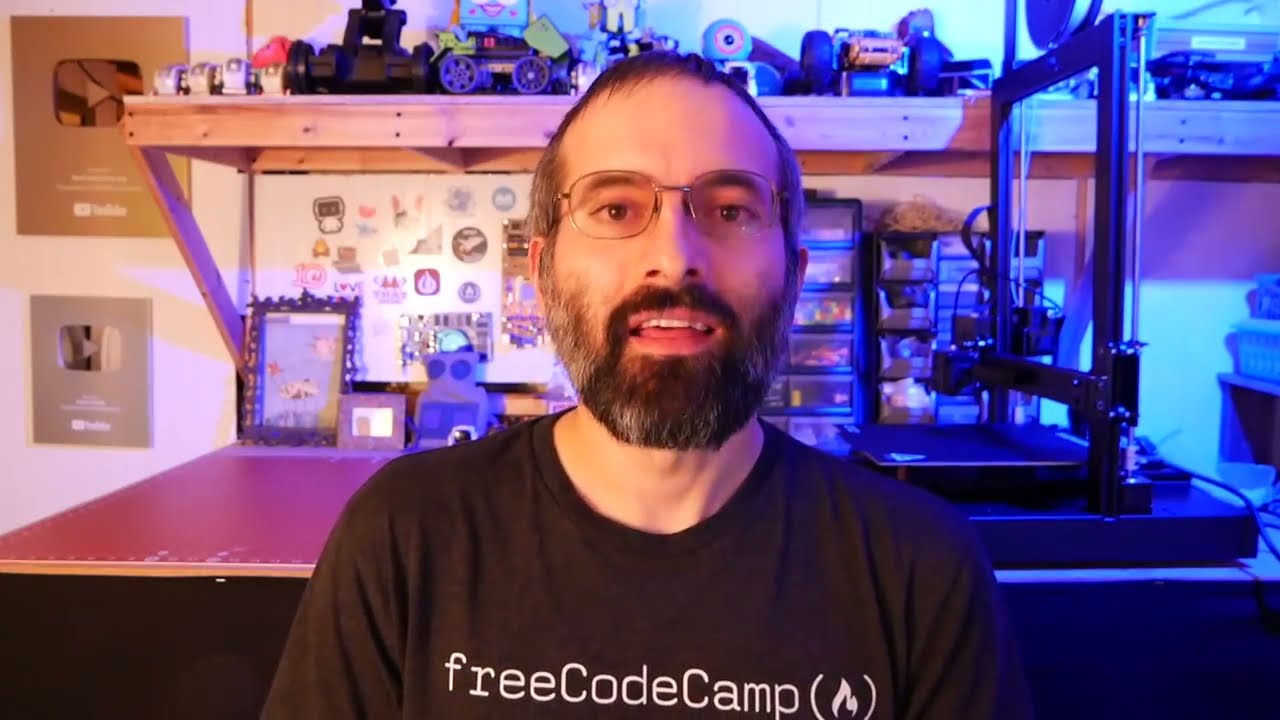
Python has gained popularity among data analysts due to its ease of use and flexibility. Let’s look at some of the reasons why Python is widely used in data analysis:
Easy to Learn and Use
One of the main advantages of using Python for data analysis is its simple syntax, which makes it easy to learn and use. Compared to other programming languages like Java or C++, Python requires fewer lines of code to perform the same task. This makes it more readable and less prone to errors, making it an ideal language for beginners.
Versatility
Python offers a wide range of libraries specifically designed for data analysis, such as Pandas, NumPy, and SciPy. These libraries provide powerful tools for data manipulation, cleaning, and analysis, making Python a versatile language for data science. Moreover, Python is not limited to data analysis; it can also be used for web development, artificial intelligence, and machine learning.
Open-Source
Another significant advantage of using Python is that it is an open-source language. This means that the source code is freely available for anyone to use, modify, and distribute. This has led to a vast community of developers continuously working on improving the language, making it more efficient and effective for data analysis.
Integration with Other Technologies
Python can easily integrate with other technologies, making it suitable for various data analytics operations. For example, it can be integrated with SQL databases to extract data for analysis or with JavaScript for creating interactive visualizations. This allows data scientists to work with different tools and technologies without having to switch between languages.
Basics of Python Programming

Before diving into data analysis techniques, it is crucial to understand the basics of Python programming. In this section, we will cover the fundamental concepts of Python that are essential for data analysis.
Variables and Data Types
Variables are containers used to store values in Python. Unlike other programming languages, you don’t need to specify the type of variable when assigning a value; Python will automatically assign the appropriate data type based on the value assigned. Some common data types in Python include integers, floats, strings, and booleans.
# Assigning variables
age = 26
# integer
height = 1.75
# float
name = "John"
# string
is_student = True
# boolean
Operators
Operators are symbols used to perform operations on variables or values. Some commonly used operators in Python include arithmetic operators (+, -, *, /), comparison operators (>, 18)
# Output: True
# Logical operators
is_student = True
is_employed = False
print(is_student and is_employed)
# Output: False
Conditional Statements
Conditional statements allow the execution of a certain block of code based on a specific condition. The most common conditional statements in Python are if, elif, and else.
# Example
age = 26
if age >= 18:
print("You are an adult")
elif age 25]
# Merging two datasets
df1 = pd.read_csv("data1.csv")
df2 = pd.read_csv("data2.csv")
merged_df = pd.merge(df1, df2, on="user_id")
Exploratory Data Analysis
Exploratory data analysis (EDA) is the process of analyzing and visualizing data to understand its characteristics and find patterns or trends. This is an essential step in data analysis as it helps identify outliers, correlations, or anomalies that may influence the results. Python provides several libraries, such as Matplotlib and Seaborn, for creating various types of plots and charts to visualize the data.
# Importing Matplotlib and Seaborn
import matplotlib.pyplot as plt
import seaborn as sns
# Creating a scatter plot
sns.scatterplot(data=df, x="age", y="salary")
# Creating a bar chart
sns.barplot(data=df, x="department", y="sales")
Statistical Analysis
Python also has libraries for performing statistical analysis on data. For example, the SciPy library provides functions for calculating descriptive statistics, hypothesis testing, and probability distributions. These libraries make it easy to perform complex statistical calculations without having to write code from scratch.
# Importing SciPy
import scipy.stats as stats
# Calculating mean and standard deviation
mean = stats.mean(df["age"])
std = stats.std(df["salary"])
# Performing t-test
group1 = df[df["department"] == "Marketing"]["sales"]
group2 = df[df["department"] == "IT"]["sales"]
t, p = stats.ttest_ind(group1, group2)
# Calculating probability
probability = stats.norm.cdf(75, 70, 5)
Machine Learning
Python is also widely used for machine learning, a subset of data science that involves training models on data to make predictions or decisions. Python provides several libraries, such as scikit-learn and TensorFlow, for implementing machine learning algorithms and creating models. These libraries offer pre-built functions for data preprocessing, model training, and evaluation, making it easier for data scientists to build and deploy machine learning models.
# Importing scikit-learn
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
from sklearn.preprocessing import StandardScaler
# Preprocessing data
X = df[["age", "experience"]]
y = df["salary"]
scaler = StandardScaler()
scaled_X = scaler.fit_transform(X)
# Splitting data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(scaled_X, y, test_size=0.2)
# Training a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
# Evaluating the model
mse = mean_squared_error(y_test, predictions)
Hands-On Python Exercises
The best way to improve your skills in Python for data analysis is to practice coding. In this section, we will provide some hands-on exercises that you can try out to test your knowledge of Python and data analysis techniques.
Exercise 1: Data Cleaning
In this exercise, you will use the Pandas library to clean a dataset and prepare it for analysis.
- Download the dataset of Pima Indians Diabetes Database from Kaggle.
- Import the necessary libraries and read the dataset into a Pandas DataFrame.
# Importing Pandas
import pandas as pd
# Reading the dataset
df = pd.read_csv("diabetes.csv")
- Check for missing values in the dataset and handle them accordingly.
# Checking for missing values
df.isnull().sum()
# Handling missing values by replacing them with the mean
df["Glucose"].fillna(df["Glucose"].mean(), inplace=True)
- Remove irrelevant columns from the dataset, such as “SkinThickness” and “Insulin”, which have many missing values and may not be useful for analysis.
# Dropping irrelevant columns
df.drop(["SkinThickness", "Insulin"], axis=1, inplace=True)
- Convert the column “Outcome” to a binary format, where “1” represents diabetes and “0” represents no diabetes.
# Converting Outcome column to binary format
df["Outcome"] = df["Outcome"].replace()
Exercise 2: Exploratory Data Analysis
In this exercise, you will use the Matplotlib and Seaborn libraries to create visualizations and explore the dataset from Exercise 1.
- Create a histogram to visualize the distribution of glucose levels in the dataset.
# Creating a histogram
plt.hist(df["Glucose"])
plt.xlabel("Glucose Levels")
plt.ylabel("Count")
- Create a scatter plot to visualize the relationship between age and BMI.
# Creating a scatter plot
plt.scatter(df["Age"], df["BMI"])
plt.xlabel("Age")
plt.ylabel("BMI")
- Create a bar chart to compare the number of people with and without diabetes in the dataset.
# Creating a bar chart
sns.countplot(data=df, x="Outcome")
plt.xlabel("Diabetes Status")
plt.ylabel("Count")
Exercise 3: Machine Learning
In this exercise, you will use the scikit-learn library to build and evaluate a machine learning model.
- Preprocess the data from Exercise 1 by splitting it into training and testing sets and scaling the features.
# Importing libraries
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
# Splitting data into features and target
X = df.drop("Outcome", axis=1)
y = df["Outcome"]
# Scaling the features
scaler = StandardScaler()
scaled_X = scaler.fit_transform(X)
# Splitting into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(scaled_X, y, test_size=0.2)
- Train a logistic regression model on the training set and make predictions on the test set.
# Importing libraries
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score
# Training the model
model = LogisticRegression()
model.fit(X_train, y_train)
# Making predictions
predictions = model.predict(X_test)
- Evaluate the model’s performance by calculating the accuracy score.
# Calculating accuracy score
accuracy = accuracy_score(y_test, predictions)
print("Accuracy:", accuracy)
Conclusion and Next Steps
In this blog post, we have explored the basics of Python programming and its importance in data analysis. We have covered some essential data analysis techniques and provided hands-on exercises to help you practice your skills. As you continue to learn and practice Python for data analysis, you can explore more advanced topics such as web scraping, natural language processing, and deep learning. With dedication and practice, you can become a master in data science and unlock endless opportunities in the job market. So what are you waiting for? Start learning Python for data analysis today!